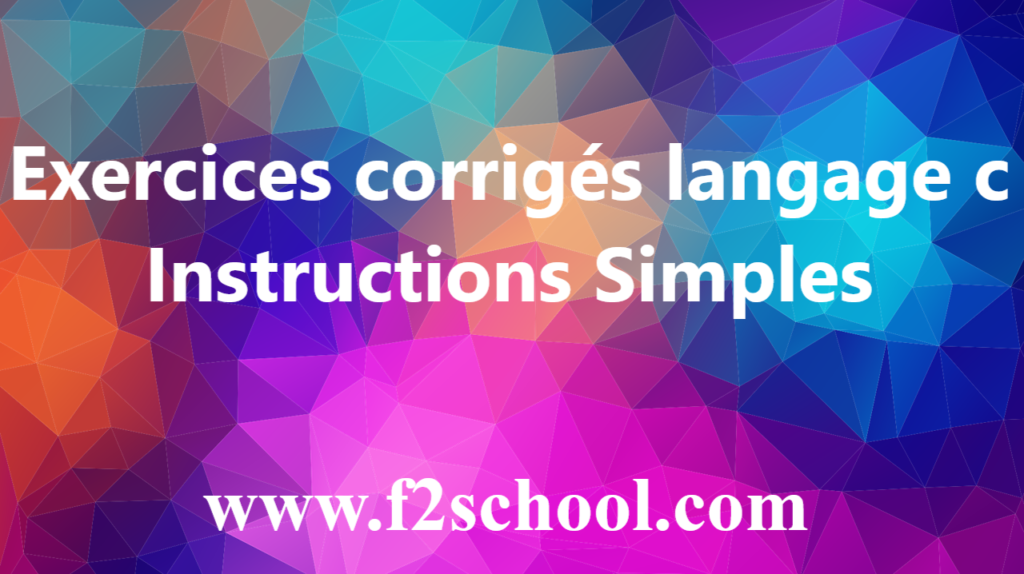
Exercices corrigés langage c – Instructions Simples
Exercice 1
Écrire un algorithme qui calcule et affiche le quotient et le reste de la division entière de deux nombres entiers saisis au clavier.
Solution
#include <stdio.h>
void main()
{
int a,b;
float rest,quot;
printf(“donner deux entiers: \n”);
scanf(“%d %d”,&a,&b);
rest=a%b;
quot=a/b;
printf(“le quotient de a/b est %.1f \n le reste de a/b est %.1f”,quot,rest);
}
Exercice 2
Écrire un algorithme qui calcule et affiche la résistance équivalente à trois résistances (dont les valeurs sont saisies au clavier) branchées en série et en parallèle. On rappelle que :
Rsérie = R1+ R2 + R3 Rparallèle= (R1+R2+R3)/(R1R2+R1R3+R2R3)
Solution
#include <stdio.h>
void main()
{
float r1,r2,r3,rs,rp;
printf(“donner trois resistances: \n”);
scanf(“%f %f %f”,&r1,&r2,&r3);
rs=r1+r2+r3;
rp=rs/((r1*r2)+(r1*r3)+(r2*r3));
printf(“Rs= %.3f \nRp= %.3f “,rs,rp);
}
Exercice 3
Écrire un algorithme qui permet d’échanger les valeurs de 3 entiers saisis au clavier (valeur de B en A, valeur de C en B, valeur de A en C).
Solution
#include <stdio.h>
void main()
{
int a,b,c,aux;
printf(“donner trois entiers: \n”);
scanf(“%d %d %d”,&a,&b,&c);
printf(“avant permutation:\n a=%d \n b=%d \n c=%d \n”,a,b,c);
aux=a;
a=c;
c=b;
b=aux;
printf(“après permutation:\n a=%d \n b=%d \n c=%d “,a,b,c);
}
Exercice 4
Un ouvrier est payé 3.397 DT par heure et 4.322 DT pour les heures supplémentaires. Il subit une retenue de base de 2.2% sur son salaire brut (le salaire brut est le salaire obtenu en tenant compte des heures de base et des heures supplémentaires).
Écrire un algorithme qui, connaissant le nombre d’heures de base et le nombre d’heures supplémentaires, permet de calculer le salaire final de l’ouvrier.
Solution
#include <stdio.h>
void main()
{
const int ph=2;
const int phs=2;
int nbh,nbhs;
float sbrut,sfinal;
printf(“donner nombre d’heures de base et supplementaire: \n”);
scanf(“%d %d”,&nbh,&nbhs);
int r=ph;
int rs=phs;
sbrut=(nbh*ph)+(nbhs*phs);
sfinal=(97.8*sbrut)/100;
printf(“Votre salaire finanl est %f “,sfinal);
}
Exercice 5
Écrire un algorithme qui permet de calculer la somme de 4 valeurs saisies au clavier
- en utilisant 5 variables
- en utilisant 2 variables
Solution
#include <stdio.h>
void main()
{
int a,b,c,d,s;
printf(“donner 4 entiers \n”);
scanf(“%d %d %d %d”,&a,&b,&c,&d);
s=a+b+c+d;
printf(“somme = %d \n”,s);
// METHODE 2
int v1,v2=0;
printf(“AUTRE METHODE DE 2 VAR : \n donner 1 entiers \n”);
scanf(“%d”,&v1);
v2=v2+v1;
printf(“donner 1 entiers \n”);
scanf(“%d”,&v1);
v2=v2+v1;
printf(“donner 1 entiers \n”);
scanf(“%d”,&v1);
v2=v2+v1;
printf(“donner 1 entiers \n”);
scanf(“%d”,&v1);
v2=v2+v1;
printf(“La somme est s= %d \n”,v2);
}
Exercice 6
Dire si le nom de ces variables est valide ou non valide.
prod_a | $total | ||
Newbal | moyenne | ||
9ab6 | _c3 | ||
c123 | new bal | ||
sum.of | grade1 | ||
Abcd | 1234 |
Solution
#include <stdio.h>
void main()
{
int prod_a;
int $total;
int Newbal;
int moyenne;
//int 9ab6; faux
int _c3;
int c123;
//int new bal; faux
//int sum.of; faux
int grade1;
int Abcd;
//int 1234; faux
Exercice 7
Écrire un algorithme qui calcule la surface d’un cercle sachant que son rayon est 2.59. Surface = rayon2 * 3.14
Solution
#include <stdio.h>
#include <math.h>
#define pi 3.14
void main()
{
const float r=2.59;
float s=pow(r,2)*pi;
//sqrt(x) racine carré
//pow(a,b) puisssance de a^b
printf(“%.3f”,s);
}
Exercice 8
Écrire un algorithme qui calcule le volume d’un cylindre sachant que son rayon est 5.2 et sa profondeur est de 2.8. Volume = rayon2 * 3.14 * profondeur.
Solution
#include <stdio.h>
#include <math.h>
#define pi 3.14
void main()
{
const float r=5.2;
const float h=2.8;
float v=pow(r,2)*pi*h;
//sqrt(x) racine carré
//pow(a,b) puisssance de a^b
printf(“%.3f”,v);
}
Exercice 9
Écrire un algorithme qui convertit une température en degré Fahrenheit en une température degré celsius. Sachant que la température en degré Fahrenheit est égale à 41. temp _cal = 5/9×(temp_far-32)
Solution
#include <stdio.h>
void main()
{
const float tmpf=62;
float tmpc;
tmpc=(5.0/9.0)*(tmpf-32);
printf(“%f”,tmpc);
}
My brother suggested I might like this blog.
He was totally right. This post truly made my day.
You can not imagine just how much time I had spent for this info!
Thanks!
I’m amazed, I have to admit. Rarely do I encounter a blog that’s both educative and interesting, and without a doubt, you have hit the
nail on the head. The issue is something that not enough people are speaking intelligently about.
I am very happy I found this during my search for
something regarding this.
I have read so many articles or reviews on the topic of the
blogger lovers except this paragraph is actually
a nice paragraph, keep it up.
Hi there, I want to subscribe for this web site to get newest updates, therefore where can i do it please help
out.
Hey there just wanted to give you a quick heads up.
The text in your content seem to be running off the screen in Safari.
I’m not sure if this is a formatting issue or something to
do with web browser compatibility but I thought I’d post to let you know.
The style and design look great though! Hope you get
the problem resolved soon. Cheers
Hi there just wanted to give you a quick heads up.
The words in your content seem to be running off the screen in Ie.
I’m not sure if this is a formatting issue or something
to do with browser compatibility but I figured
I’d post to let you know. The design look great though!
Hope you get the issue fixed soon. Kudos
This is very interesting, You’re a very skilled blogger.
I have joined your rss feed and look forward to seeking more
of your great post. Also, I’ve shared your site in my social networks!
Attractive component of content. I simply stumbled upon your blog and in accession capital to assert that I get in fact loved
account your weblog posts. Anyway I will be subscribing on your feeds and even I fulfillment you access persistently quickly.
Quality articles or reviews is the main to attract
the people to go to see the web page, that’s what this web site is providing.
Hi there just wanted to give you a quick heads up. The
words in your article seem to be running off the screen in Safari.
I’m not sure if this is a formatting issue or something to do with browser compatibility but I figured I’d
post to let you know. The design and style look great though!
Hope you get the issue resolved soon. Many thanks
What’s up i am kavin, its my first occasion to commenting anywhere, when i read this article i thought i could also make comment due to this brilliant paragraph.
Hi there it’s me, I am also visiting this web page on a
regular basis, this web site is in fact good and the people are in fact sharing pleasant thoughts.
If some one wants expert view on the topic of blogging afterward i
suggest him/her to pay a visit this blog, Keep up the nice job.
I’m extremely inspired with your writing abilities
as well as with the format on your blog. Is that this a
paid topic or did you modify it yourself? Anyway stay up the nice quality writing, it’s rare to peer a nice blog like this one today..
Appreciating the time and energy you put into your site and in depth
information you present. It’s nice to come across a blog every once
in a while that isn’t the same outdated rehashed material.
Great read! I’ve bookmarked your site and I’m adding your
RSS feeds to my Google account.
Way cool! Some extremely valid points! I appreciate you writing this post
and the rest of the website is also really good.